The input manager class, provides fundamental functionality for work in all fields on this library.
More...
#include <ceInputCoreImpl.h>
List of all members.
Public Member Functions |
const short | VerifyInputManager (void) const |
| Verify the integrity of the manager class.
|
short | SetInputDriver (const EInputDriver driver=InputDriver_Null) const |
| Set a new driver for all devices.
|
EInputDriver | GetDriverType (void) const |
| Get the current driver type.
|
IInputDevice * | GetInputDevice (const EInputDevice device) const |
| Get the device base class from any device.
|
void | Update (void) const |
| Update the status of all devices.
|
IKeyboardDevice * | GetKeyboardDevice (void) const |
| Get the keyboard manager.
|
void | UpdateKeyboar (void) const |
| Update the status of the keyboard device.
|
IMouseDevice * | GetMouseDevice (void) const |
| Get the mouse manager.
|
void | UpdateMouse (void) const |
| Update the status of the mouse device.
|
IJoystickDevice * | GetJoystickDevice (void) const |
| Get the joystick manager.
|
void | UpdateJoystick (void) const |
| Update the status of the joystick device.
|
Protected Member Functions |
virtual void | OnKeyPressed (const IKeyboardDevice *Owner, const int key, const int buffer[256])=0x0 |
| This callback is called when a key is pressed.
|
virtual void | OnKeyReleased (const IKeyboardDevice *Owner, const int key, const int buffer[256])=0x0 |
| This callback is called when a key is released.
|
virtual void | OnKeyEvent (const IKeyboardDevice *Owner, const int buffer[256])=0x0 |
| This callback is always called while is keep pressed any key.
|
virtual void | OnButtonPressed (const IMouseDevice *Owner, const int button, const SMouseState &State)=0 |
| This callback is called when a button is pressed.
|
virtual void | OnButtonReleased (const IMouseDevice *Owner, const int button, const SMouseState &State)=0 |
| This callback is called when a button is released.
|
virtual void | OnMouseEvent (const IMouseDevice *Owner, const SMouseState &State)=0 |
| This callback is always called, when the cursor is moved or while is keep pressed any button.
|
virtual void | OnJoyButtonPressed (const IJoystickDevice *Owner, const int joyid, const unsigned int button, const SJoystickState &State)=0 |
| This callback is called when a button is pressed.
|
virtual void | OnJoyButtonReleased (const IJoystickDevice *Owner, const int joyid, const unsigned int button, const SJoystickState &State)=0 |
| This callback is called when a button is released.
|
virtual void | OnJoystickEvent (const IJoystickDevice *Owner, const int joyid, const SJoystickState &State)=0 |
| This callback is always called while is keep pressed any button or axis.
|
Protected Attributes |
IInputManager * | Input |
| Instance to the input manager.
|
IKeyboardDevice * | Keyboard |
| Instance to the keyboard manager.
|
IMouseDevice * | Mouse |
| Instance to the mouse manager.
|
IJoystickDevice * | Joystick |
| Instance to the joystick manager.
|
Detailed Description
template<class T>
class ce::IInputManagerImpl< T >
The input manager class, provides fundamental functionality for work in all fields on this library.
Member Function Documentation
const short VerifyInputManager |
( |
void |
|
) |
const [inline] |
Verify the integrity of the manager class.
- Returns:
- If this succeeds, the return is a TRUE value or if this fails, the return is a FALSE value.
short SetInputDriver |
( |
const EInputDriver |
driver = InputDriver_Null |
) |
const [inline] |
Set a new driver for all devices.
- Parameters:
-
| driver | New driver to change. |
- See also:
- GetDriverType
Get the current driver type.
- Returns:
- The current driver type.
- See also:
- SetInputDriver
Get the device base class from any device.
- Returns:
- The device base class.
void Update |
( |
void |
|
) |
const [inline] |
Update the status of all devices.
void UpdateKeyboar |
( |
void |
|
) |
const [inline] |
Update the status of the keyboard device.
void UpdateMouse |
( |
void |
|
) |
const [inline] |
Update the status of the mouse device.
void UpdateJoystick |
( |
void |
|
) |
const [inline] |
Update the status of the joystick device.
virtual void OnKeyPressed |
( |
const IKeyboardDevice * |
Owner, |
|
|
const int |
key, |
|
|
const int |
buffer[256] | |
|
) |
| | [pure virtual, inherited] |
This callback is called when a key is pressed.
- Parameters:
-
| Owner | The keyboard manager owner. |
| key | The virtual key was pressed. If the desired virtual key is a letter or digit (A through Z, a through z, or 0 through 9), key must be set to the ASCII value of that character. For other keys, it must be a virtual-key code. |
| buffer | Pointer to a 256-byte array that contains keyboard key states. |
- See also:
- OnKeyReleased, OnKeyEvent
virtual void OnKeyReleased |
( |
const IKeyboardDevice * |
Owner, |
|
|
const int |
key, |
|
|
const int |
buffer[256] | |
|
) |
| | [pure virtual, inherited] |
This callback is called when a key is released.
- Parameters:
-
| Owner | The keyboard manager owner. |
| key | The virtual key was pressed. If the desired virtual key is a letter or digit (A through Z, a through z, or 0 through 9), key must be set to the ASCII value of that character. For other keys, it must be a virtual-key code. |
| buffer | Pointer to a 256-byte array that contains keyboard key states. |
- See also:
- OnKeyPressed, OnKeyEvent
virtual void OnKeyEvent |
( |
const IKeyboardDevice * |
Owner, |
|
|
const int |
buffer[256] | |
|
) |
| | [pure virtual, inherited] |
This callback is always called while is keep pressed any key.
- Parameters:
-
| Owner | The keyboard manager owner. |
| buffer | Pointer to a 256-byte array that contains keyboard key states. |
- See also:
- OnKeyPressed, OnKeyReleased
virtual void OnButtonPressed |
( |
const IMouseDevice * |
Owner, |
|
|
const int |
button, |
|
|
const SMouseState & |
State | |
|
) |
| | [pure virtual, inherited] |
This callback is called when a button is pressed.
- Parameters:
-
| Owner | The mouse manager owner. |
| button | The button was pressed. |
| State | The mouse state. |
- See also:
- OnButtonReleased, OnMouseEvent
virtual void OnButtonReleased |
( |
const IMouseDevice * |
Owner, |
|
|
const int |
button, |
|
|
const SMouseState & |
State | |
|
) |
| | [pure virtual, inherited] |
This callback is called when a button is released.
- Parameters:
-
| Owner | The mouse manager owner. |
| button | The button was released. |
| State | The mouse state. |
- See also:
- OnButtonPressed, OnMouseEvent
This callback is always called, when the cursor is moved or while is keep pressed any button.
- Parameters:
-
| Owner | The mouse manager owner. |
| State | The mouse state. |
- See also:
- OnButtonPressed, OnButtonReleased
virtual void OnJoyButtonPressed |
( |
const IJoystickDevice * |
Owner, |
|
|
const int |
joyid, |
|
|
const unsigned int |
button, |
|
|
const SJoystickState & |
State | |
|
) |
| | [pure virtual, inherited] |
This callback is called when a button is pressed.
- Parameters:
-
| Owner | The joystick manager owner. |
| joyid | The joystick id. |
| button | The button was pressed. |
| State | The joystick state. |
- See also:
- OnJoyButtonReleased, OnJoystickEvent
virtual void OnJoyButtonReleased |
( |
const IJoystickDevice * |
Owner, |
|
|
const int |
joyid, |
|
|
const unsigned int |
button, |
|
|
const SJoystickState & |
State | |
|
) |
| | [pure virtual, inherited] |
This callback is called when a button is released.
- Parameters:
-
| Owner | The joystick manager owner. |
| joyid | The joystick id. |
| button | The button was pressed. |
| State | The joystick state. |
- See also:
- OnJoyButtonPressed, OnJoystickEvent
This callback is always called while is keep pressed any button or axis.
- Parameters:
-
| Owner | The joystick manager owner. |
| joyid | The joystick id. |
| State | The joystick state. |
- See also:
- OnJoyButtonPressed, OnJoyButtonReleased
Member Data Documentation
Instance to the input manager.
Instance to the keyboard manager.
Instance to the mouse manager.
Instance to the joystick manager.
The documentation for this class was generated from the following file:
|
|
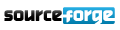 |
|