The mouse device, this is the mouse manager.
More...
#include <ceMouse.h>
List of all members.
Public Member Functions |
virtual int | IsButtonPressed (const int button=0x0) const =0 |
| Check whether a button is pressed, at any given time.
|
virtual int | IsLButtonPressed (void) const =0 |
| Check whether left button is pressed, at any given time.
|
virtual int | IsRButtonPressed (void) const =0 |
| Check whether right button is pressed, at any given time.
|
virtual int | IsMButtonPressed (void) const =0 |
| Check whether middle button is pressed, at any given time.
|
virtual int | IsButtonReleased (const int button=0x0) const =0 |
| Check whether a button is released, at any given time.
|
virtual int | IsLButtonReleased (void) const =0 |
| Check whether left button is released, at any given time.
|
virtual int | IsRButtonReleased (void) const =0 |
| Check whether right button is released, at any given time.
|
virtual int | IsMButtonReleased (void) const =0 |
| Check whether middle button is released, at any given time.
|
virtual int | GetPosition (unsigned int *get_x, unsigned int *get_y) const =0 |
| Retrieves the cursor's position, in screen coordinates.
|
virtual unsigned int | GetXPosition (void) const =0 |
| Retrieves the X position of the cursor, in screen coordinates.
|
virtual unsigned int | GetYPosition (void) const =0 |
| Retrieves the Y position of the cursor, in screen coordinates.
|
virtual void | SetMouseEvent (IMouseEvent *Event) const =0 |
| Set the mouse event.
|
virtual IMouseEvent * | GetMouseEvent (void) const =0 |
| Retrieves the mouse event.
|
virtual EInputDevice | GetDeviceType (void) const =0 |
| Retrieves the device type.
|
virtual char * | GetDeviceName (void) const =0 |
| Retrieves the device name.
|
virtual int | GetDeviceCount (void) const =0 |
| Retrieves the device count.
|
virtual int | IsDevicePresent (const int id=0x0) const =0 |
| Check whether a device is present, at any given time.
|
virtual void | Update (const int async=TRUE)=0 |
| Update status of only this device.
|
Detailed Description
The mouse device, this is the mouse manager.
- See also:
- IMouseEvent
- Todo:
- Methods:
- AttachMouseEvent(IMouseEvent *Event) : short(index)
- DetachMouseEvent(IMouseEvent *Event)
- GetMouseEvent(const short index)
Member Function Documentation
virtual int IsButtonPressed |
( |
const int |
button = 0x0 |
) |
const [pure virtual] |
Check whether a button is pressed, at any given time.
- Parameters:
-
| button | The button to check. |
- Returns:
- If the button is pressed, the return is a TRUE value or if the button is released, the return is a FALSE value.
- See also:
- IsButtonReleased
virtual int IsLButtonPressed |
( |
void |
|
) |
const [pure virtual] |
Check whether left button is pressed, at any given time.
- Returns:
- If the button is pressed, the return is a TRUE value or if the button is released, the return is a FALSE value.
virtual int IsRButtonPressed |
( |
void |
|
) |
const [pure virtual] |
Check whether right button is pressed, at any given time.
- Returns:
- If the button is pressed, the return is a TRUE value or if the button is released, the return is a FALSE value.
virtual int IsMButtonPressed |
( |
void |
|
) |
const [pure virtual] |
Check whether middle button is pressed, at any given time.
- Returns:
- If the button is pressed, the return is a TRUE value or if the button is released, the return is a FALSE value.
virtual int IsButtonReleased |
( |
const int |
button = 0x0 |
) |
const [pure virtual] |
Check whether a button is released, at any given time.
- Parameters:
-
| button | The button to check. |
- Returns:
- If the button is released, the return is a TRUE value or if the button is pressed, the return is a FALSE value.
- See also:
- IsButtonPressed
virtual int IsLButtonReleased |
( |
void |
|
) |
const [pure virtual] |
Check whether left button is released, at any given time.
- Returns:
- If the button is released, the return is a TRUE value or if the button is pressed, the return is a FALSE value.
virtual int IsRButtonReleased |
( |
void |
|
) |
const [pure virtual] |
Check whether right button is released, at any given time.
- Returns:
- If the button is released, the return is a TRUE value or if the button is pressed, the return is a FALSE value.
virtual int IsMButtonReleased |
( |
void |
|
) |
const [pure virtual] |
Check whether middle button is released, at any given time.
- Returns:
- If the button is released, the return is a TRUE value or if the button is pressed, the return is a FALSE value.
virtual int GetPosition |
( |
unsigned int * |
get_x, |
|
|
unsigned int * |
get_y | |
|
) |
| | const [pure virtual] |
Retrieves the cursor's position, in screen coordinates.
- Parameters:
-
| get_x | A pointer that receives the X position of the cursor. |
| get_y | A pointer that receives the Y position of the cursor. |
- Returns:
- if this succeeds, the return is a TRUE value or if this fails, the return is a FALSE value.
virtual unsigned int GetXPosition |
( |
void |
|
) |
const [pure virtual] |
Retrieves the X position of the cursor, in screen coordinates.
- Returns:
- The X position of the cursor.
virtual unsigned int GetYPosition |
( |
void |
|
) |
const [pure virtual] |
Retrieves the Y position of the cursor, in screen coordinates.
- Returns:
- The Y position of the cursor.
virtual void SetMouseEvent |
( |
IMouseEvent * |
Event |
) |
const [pure virtual] |
Set the mouse event.
- Parameters:
-
| Event | Pointer to mouse event. |
virtual IMouseEvent* GetMouseEvent |
( |
void |
|
) |
const [pure virtual] |
Retrieves the mouse event.
- Returns:
- The mouse event.
virtual EInputDevice GetDeviceType |
( |
void |
|
) |
const [pure virtual, inherited] |
Retrieves the device type.
- Returns:
- The device type.
virtual char* GetDeviceName |
( |
void |
|
) |
const [pure virtual, inherited] |
Retrieves the device name.
- Returns:
- The device name.
virtual int GetDeviceCount |
( |
void |
|
) |
const [pure virtual, inherited] |
Retrieves the device count.
- Returns:
- The number of devices attached.
virtual int IsDevicePresent |
( |
const int |
id = 0x0 |
) |
const [pure virtual, inherited] |
Check whether a device is present, at any given time.
- Returns:
- If this succeeds, the return is a TRUE value or if this fails, the return is a FALSE value.
virtual void Update |
( |
const int |
async = TRUE |
) |
[pure virtual, inherited] |
Update status of only this device.
- Parameters:
-
| async | Ignore this parameter. |
The documentation for this class was generated from the following file:
|
|
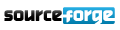 |
|